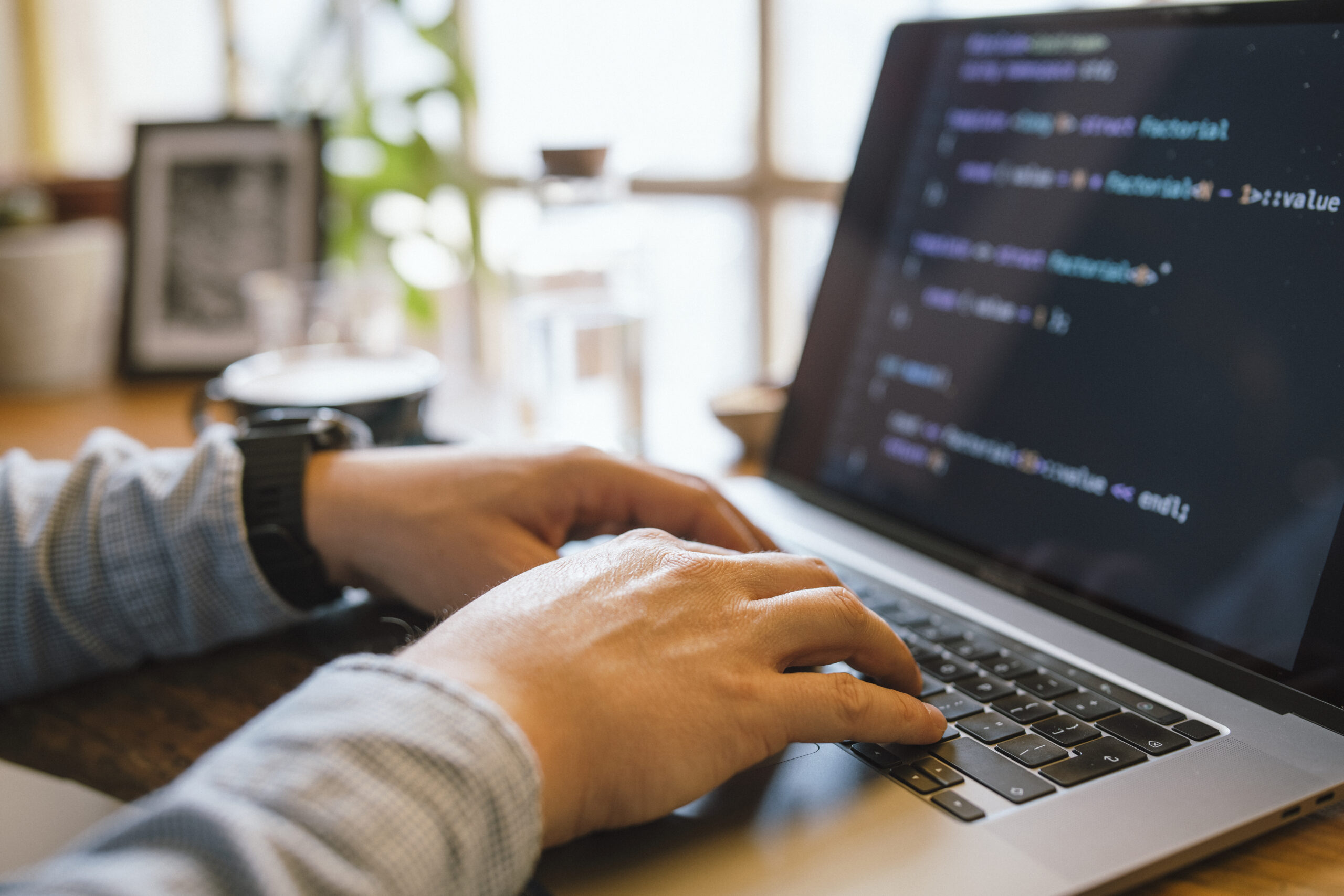
Debugging is Just about the most vital — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about comprehending how and why issues go Improper, and Finding out to Consider methodically to resolve troubles successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and significantly enhance your productivity. Here are several procedures that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest approaches developers can elevate their debugging abilities is by mastering the applications they use on a daily basis. When composing code is a single A part of development, recognizing the way to interact with it effectively all through execution is equally vital. Modern-day advancement environments come Geared up with strong debugging capabilities — but quite a few developers only scratch the area of what these equipment can do.
Acquire, by way of example, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code over the fly. When utilised correctly, they Allow you to notice specifically how your code behaves during execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, see authentic-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or technique-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about running processes and memory management. Mastering these tools might have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with Edition Handle devices like Git to be familiar with code history, locate the exact second bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools indicates heading over and above default settings and shortcuts — it’s about building an personal familiarity with your progress ecosystem so that when problems arise, you’re not lost at midnight. The better you realize your resources, the more time you are able to invest solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Ahead of jumping into the code or making guesses, builders need to have to create a consistent ecosystem or circumstance the place the bug reliably appears. Without reproducibility, fixing a bug results in being a video game of possibility, frequently leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting just as much context as is possible. Request questions like: What steps led to The difficulty? Which surroundings was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it gets to isolate the exact ailments below which the bug takes place.
After you’ve gathered adequate information, try and recreate the issue in your neighborhood environment. This might mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the edge scenarios or state transitions concerned. These checks not only support expose the condition but additionally protect against regressions in the future.
Often, The difficulty may be surroundings-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is usually instrumental in replicating such bugs.
Reproducing the trouble isn’t simply a step — it’s a frame of mind. It necessitates patience, observation, along with a methodical strategy. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible scenario, You should use your debugging resources much more efficiently, examination prospective fixes securely, and talk a lot more Obviously along with your crew or consumers. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders prosper.
Go through and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most precious clues a developer has when one thing goes Improper. Instead of seeing them as frustrating interruptions, builders really should understand to deal with error messages as immediate communications through the program. They frequently tell you what precisely took place, in which it happened, and sometimes even why it took place — if you understand how to interpret them.
Begin by examining the concept very carefully As well as in whole. A lot of developers, specially when underneath time strain, glance at the 1st line and quickly begin earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into search engines — study and have an understanding of them 1st.
Break the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These concerns can tutorial your investigation and stage you toward the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Error messages in languages like Python, JavaScript, or Java normally adhere to predictable designs, and learning to recognize these can considerably speed up your debugging approach.
Some faults are vague or generic, and in People conditions, it’s vital to look at the context wherein the error occurred. Check out similar log entries, input values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger challenges and provide hints about possible bugs.
Eventually, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint concerns more rapidly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most effective resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, Data, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details in the course of improvement, INFO for typical situations (like prosperous start off-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. An excessive amount logging can obscure critical messages and slow down your procedure. Center on crucial events, point out adjustments, enter/output values, and significant choice details within your code.
Structure your log messages Obviously and regularly. Involve context, for example timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t feasible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. Using a very well-thought-out logging technique, you could reduce the time it will require to identify troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a form of investigation. To successfully discover and deal with bugs, builders must tactic the process like a detective solving a mystery. This frame of mind can help stop working elaborate issues into manageable elements and comply with clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or overall performance concerns. Identical to a detective surveys against the law scene, obtain just as much suitable facts as you could without the need of leaping to conclusions. Use logs, exam scenarios, and person stories to piece collectively a clear image of what’s happening.
Next, form hypotheses. Talk to you: What can be resulting in this habits? Have any alterations just lately been created towards the codebase? Has this issue happened in advance of beneath equivalent situations? The goal should be to slim down prospects and determine potential culprits.
Then, exam your theories systematically. Try and recreate the trouble in a managed surroundings. When you suspect a certain perform or component, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code queries and let the final results lead you nearer to the truth.
Pay shut interest to compact information. Bugs frequently disguise inside the least predicted locations—similar to a missing semicolon, an off-by-one particular error, or a race issue. Be thorough and client, resisting the urge to patch the issue devoid of totally being familiar with it. Short term fixes may perhaps conceal the actual issue, just for it to resurface afterwards.
And lastly, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other folks have an understanding of your reasoning.
By pondering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become simpler at uncovering concealed difficulties in complex techniques.
Publish Checks
Writing tests is one of the best strategies to help your debugging skills and General growth effectiveness. Assessments don't just help catch bugs early but additionally serve as a safety net that gives you self-assurance when generating improvements towards your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint precisely exactly where and when an issue occurs.
Start with unit checks, which focus on individual capabilities or modules. These compact, isolated checks can promptly expose no matter if a certain bit of logic is Doing work as anticipated. Whenever a test fails, you immediately know where to glimpse, noticeably cutting down enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after previously being preset.
Following, integrate integration tests and conclusion-to-conclude exams into your workflow. These help make sure several elements of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complex methods with various elements or services interacting. If a little something breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Producing exams also forces you to definitely Feel critically regarding your code. To test a aspect effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of comprehension naturally qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a strong starting point. After the take a look at fails consistently, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This approach ensures that a similar bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing match right into a structured and predictable process—assisting you catch additional bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the problem—looking at your display for hrs, striving Option just after Alternative. But Probably the most underrated debugging resources is just stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and sometimes see The problem from a new perspective.
When you're as well close to the code for too long, cognitive fatigue sets in. You might start overlooking obvious faults or misreading code that you choose to wrote just several hours previously. In this particular condition, your brain gets to be less efficient at trouble-resolving. A brief stroll, a coffee crack, or maybe switching to a unique activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the background.
Breaks also help protect against burnout, Specially in the course of lengthier debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Strength along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to maneuver close to, extend, or do one thing unrelated to code. It may well really feel counterintuitive, Primarily below limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging In the long term.
In short, getting breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your point of view, and helps you avoid the tunnel eyesight That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you come across is much more than simply A short lived setback—It is a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can teach you one thing worthwhile when you take the time to reflect and analyze what went Improper.
Start off by inquiring on your own some vital questions once the bug is resolved: What brought on it? Why did it go unnoticed? Could it happen to be caught earlier with far better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or understanding and help you Develop stronger coding routines moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log where you Be aware down bugs you’ve encountered, how you solved them, and what you learned. Eventually, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends could be Particularly impressive. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, some of the ideal developers will not be the ones who compose fantastic code, but individuals who consistently find out from their issues.
Ultimately, Each individual bug you resolve provides a new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll appear away a smarter, a lot more able developer due to it.
Conclusion
Improving upon your debugging abilities normally takes time, observe, and patience — even so the payoff is large. It makes you a more successful, self-assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging more info isn’t a chore — it’s a possibility to be better at Everything you do.